Creating a new Project in 7 steps with .Net MVC & Visual Studio Part 1
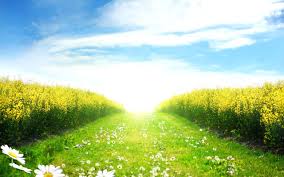
Over the next 2 posts I will be creating a starting point for a .Net MVC Application. The first post will concentrate on creating an empty project with a structure that can be used to build an application using your own preferences for technologies and packages. During the second post I will be installing some of the packages that I commonly use when I’m developing an application. I’ve tried this layout in a couple of applications and I’ve learned what I like to use, but I regularly re-evaluate these technologies to make sure that there isn’t something that has come along that will meet my needs better.
- Entity Framework Code First
- Fluent
- Ninject
- N-Unit
- Logger
- Identity V2
Entity Framework allows me to concentrate on writing C# code and designing my applications without having to take time to write SQL. I like this because I can concentrate on developing the application and I trust Entity Framework to look after the SQL code needed to build the database. The main aspects I find useful is that I can easily re-build the database as needed, rolling back the database is trivial, and changing the structure of the database is managed for me automatically because it generates SQL code for me based of the Models project.
Fluent is one of the ways you can configure your database, the other way is using Data Annotations. Fluent is a little bit harder to use than Data Annotations but it gives me more control over the database without making my Model classes messy with multiple annotations per object. This makes it easier to read what is in the model.
Ninject is for dependency injection. I use it because it helps make my code easy to test. There are plenty of dependency injection packages out there, I use Ninject because I find it straight to configure and I haven’t had any problems with it. Using dependency Injection allows me to easily use interfaces in my code rather than instances of types. A full description of Ninject and its features are available at http://www.ninject.org/ . They also use ninjas and that’s pretty cool.
N-Unit again I use this because I like it, it was recommended to me when I started developing .Net applications over the Microsoft test framework and I’ve stuck with it since. It was easy to pick up coming from Java development in university using J-Unit. This is because it is based off the same X-Unit framework. A great resource for N-Unit is Roy Osherov http://osherove.com/ .
Logger is used to log application errors to the database. Simple really. It will log any server side error to a database automatically, it is easy to configure and you can set the level of logging that you want. It is also easy to extend to start logging you r own application events. The earlier you implement this the better because you can log events as you are developing rather than trying to go back over your application and remember all the events that happen.
Identity V2 this is used for authentication and security. It is a pretty detailed package and it is very flexible. There is a lot to learn about this and it can be a little tricky to set up initially. The best place to get started with this is by setting up a sample application from Visual Studio that has Identity set up for you. This will allow you to play with it and see how it works. I will follow the structure from the sample app, it is pretty well laid out and I see no reason to change it.
All of these packages are available through Nuget package manager, I like using NuGet because it provides an easy way to search and manage the packages for an application.
The rest of this post will be pretty much a Cook Book. We will take advantage of .Nets ability to split a Solution into separate projects. The Model and Database configuration will be split into separate projects from the web application so that we can re-use them. For example if you want to add an API to your solution you can re-use the existing data structures without affecting your MVC application. This will also allow you to use separate security configurations for the API and the Web application.
Step 1: Create an Empty Project
Choose ‘ASP.Net Web Application’
Name your application any thing you want, I chose Blank Space after I heard the Taylor Swift song. It seemed suitable for what I wanted to create.
If you want performance data on you application select ‘Add Application Insights to Project’. I’ve never used this service so I can’t comment on it, but gathering this information is important especially if you are trying to debug performance issues.
Tick ‘Create directory for solution’ and if you want to use source control which I highly recommend Tick ‘Add to Source Control’. Source control has saved my ass many times.
Finally press ‘OK’
We will create an ‘Empty’ project, this is a basic skeleton for an application with no sample code like Models, Views or Controllers. You don’t want these added because they just clutter up the application and it will take more work to remove them or alter them than is needed to create new objects.
Then we will use the folders and core references for MVC structure as our starting point.
At this time we won’t tick ‘Add unit tests’ because we want to use N-Unit at a later stage. Adding Unit tests at this stage will add the standard Microsoft Testing framework. If you want it is ok to use this having any unit test framework is better than no test framework. N-unit was recommended to be and I’ve never felt the need to learn anything else. At the end of they day I want to spend my time writing applications. I tend to view Unit tests just as a tool. Using them does give you confidence in your application especially after any refactoring.
Step 2: Version Control
The next screen that you will see is ‘Choose Source Control’ you can’t go wrong with either choice. Team Foundation Version Control comes with a lot of excellent tools for managing your project with tasks and Agile management tools. These all integrate with Visual Studio and it means you can manage your entire project from one place. It also allows you to easily integrate with the Visual Studio build controller but I’m not a big fan of it but it is better than no build controller..
If you want to share your code on Git Hub or have more control over what tools you use Git might be better for you.
For this post I won’t use source control because I want to create a project template that can be used with either TFVC or Git. It is possible to switch between them but there is a bit of a work involved, I recommend thinking about what you want and pick one and stick with it for the project.
After you setup your project for source control I would recommend using a service that stores your application remotely. This allows you to easily work with others on your project and if anything happens to your local machine you will have a backup somewhere in the cloud. For Git, Git Hub is an excellent choice. There are private repositories available for pretty cheap if you don’t want to share your code publicly. If you want to stick with the integration for Microsoft products the Azure platform is one of the best Platforms as a Service (PaaS) that I have used. It is roughly the same price as Amazon, but is easier to use and set up. Although this is at the expense of the flexibility that Amazon offers.
Step 3: Rename Models
After setting up your source control, you will have something that looks a little like this.
This is the standard .Net MVC project layout. The first thing to notice is the Models folder. Earlier we said that we would be moving Models into it’s own project.
Rather than delete the folder, rename it to ‘ViewModels’. The View Models will be used to combine data from different models and pass them to our Views. This is a handy way of passing data to and from the view. This can allow the user to enter data through forms or for us to display data from the database to our users.
In Razor there are many HTML helpers available. These helpers can be used to generate forms to input data to populate the View Model or to display data from the View Model to the user. A complete list of these can be found on MSDN https://msdn.microsoft.com/en-us/library/system.web.mvc.htmlhelper_methods(v=vs.118).aspx
Step 4: Create a new Project for your Models
Now we will create the Models Project.
This will be used for Plain Old C# Objects ( POCO ). We aren’t going to use data annotations because they can get pretty ugly. Later on we will create another project to look after our database configurations.
Right Click on the solution, then look for ‘Add’ and Right Click on ‘New Project’
Under the Menu ‘Visual C#’ choose ‘Class Library’ and Name the project.
I like to follow the naming structure { solution name }.{ module } so that’s why I created the name ‘BlankSpace.Models’.
If you look in your solution now, you will notice that your original projects name is in bold compared to the new project. This is because it is the start-up project. When you run the solution this is the project that runs.
Now would be a good time to go back and rename the original MCV project as ‘BlankSpace.Web’ because this is were all our web based code will be placed.
Step 5: Create a Project to communicate with your database
We will now create another project for communicating with our database. I tend to call this DataAccess, but you can call it what ever you want, so long as it is clear that this project will communicate with the database server. So my project name will be ‘BlankSpace.DataAccess’. You can create this project in exactly the same way you created the Models project.
Step 6: Link the projects together
We need to add references for BlankSpace.DataAccess and BlankSpace.Models to BlankSpace.Web.
To do this browse to your start-up project (.Web) and Right Click on ‘References’ and choose ‘Add Reference’
Under ‘Solution’ choose ‘Projects’.
Tick both DataAccess and Models
Press ‘OK’
BlankSpace.Web now knows that the other 2 projects exist. You can now reference and use the classes from these projects. You need to do the same process and add your Models project as a Reference to the DataAccess project. Start by Right Clicking on ‘References’ in the DataAccess project.
Step 7: Build Order & Dependencies
Now we need to tell the solution what projects need to be built together and in what order. This is generally done automatically for us after we add the references. But it is good to understand how to change it or set it. For exampl it might not get set for you for some reason or some one might accidentally change it.
If you want to check, my dependencies for BlankSpace are as follows
BlankSpace.Web
- BlankSpace.Models
- BlankSpace.DataAccess
BlankSpace.Models
- None
BlankSpace.DataAccess
- BlankSpace.Models
These can be set by Right Clicking on ‘Solution’ then selecting ‘Project Dependencies…’
Then Choose the project and tick the required projects. You can check the build order but this will be set automatically by the project dependencies. The build order should be as shown in the image.
Now would be a good time to save your solution in source control if you want a pure base that can be used to experiment with different technologies and packages. From here on I will be installing packages that I like to use when I’m developing a web based application. Here is the link to my repository https://github.com/DerailedJeff/BlankSpace/tree/master/Pure/BlankSpace
In the next post I will go through installing some useful packages for the development.